here it is: An edLeak Python client is now available. It has all features of the html client (and even more), but required 6 times less lines of code.
Why a Python Client ?
Until now, the edLeak client was written in Html/Js. This choice was driven by two facts : Any OS has a decent enough web browser, and some libraries make it quite easy to write a web UI. However even though JQuery, Highcharts, and D3js help a lot, writing a decent GUI (i.e. easy to use and allowing advanced features) is still a challenge : The call stack support only required to add a button but the upcoming lifecycle feature will be more challenging.
This is where a CLI becomes an obvious choice: Adding a feature is much faster and one can improve the existing tool by writing new scripts. However some flexible plotting capabilities must be available so that the edKit visualization base principle is still available. Fortunately there is an existing python project perfectly matching the requirements of an edKit client : SciPy. SciPy is a set of python tools for mathematics and science usage. Its matplotlib library allows to plot the same graphs than the ones of the html client.
Another major advantage of a CLI interface is that it make automation leak detection possible : A leak detection script can be written and integrated in a continuous integration framework such as Jenkins so that new memory leaks are automatically detected. Even though this is not possible yet, this is one of the aims of edLeak.
A First Example
An EdLeak example is available in examples/edleak1.py. It dumps one memory slice each second during 1 minute. To test it, first run the leaker tool:
make leaker_run
Then the sample can be run from ipython:
%run examples/edleak1.py
Or by copy/pasting its code in the interpreter:
import rpc.ws import edleak.api import edleak.slice_runner import edleak.plot ws_rpc = rpc.ws.WebService("localhost", 8080) el = edleak.api.EdLeak(ws_rpc) runner = edleak.slice_runner.SliceRunner(el) asset = runner.run(1, 60) elplot = edleak.plot.SlicePlotter(asset) elplot.sizePlot()
5 object types are used here:
- WebService inherits from Rpc. This class abstracts the RPC mechanism used to access the target. In this example we use the edleak web service APIs.
- EdLeak is the object exposing the available edleak APIs.
- SliceRunner is a facility class dumping memory slices and returning an asset once completed.
- An Asset holds an edleak context : A slice list, the allocers list. Assets can be saved and loaded to/from a file.
- SlicePlotter plots some slice graphs. This class uses matplotlib to generate the plots.
The size plot used in the example is the same than the one available with the html client. The following plot is then generated :
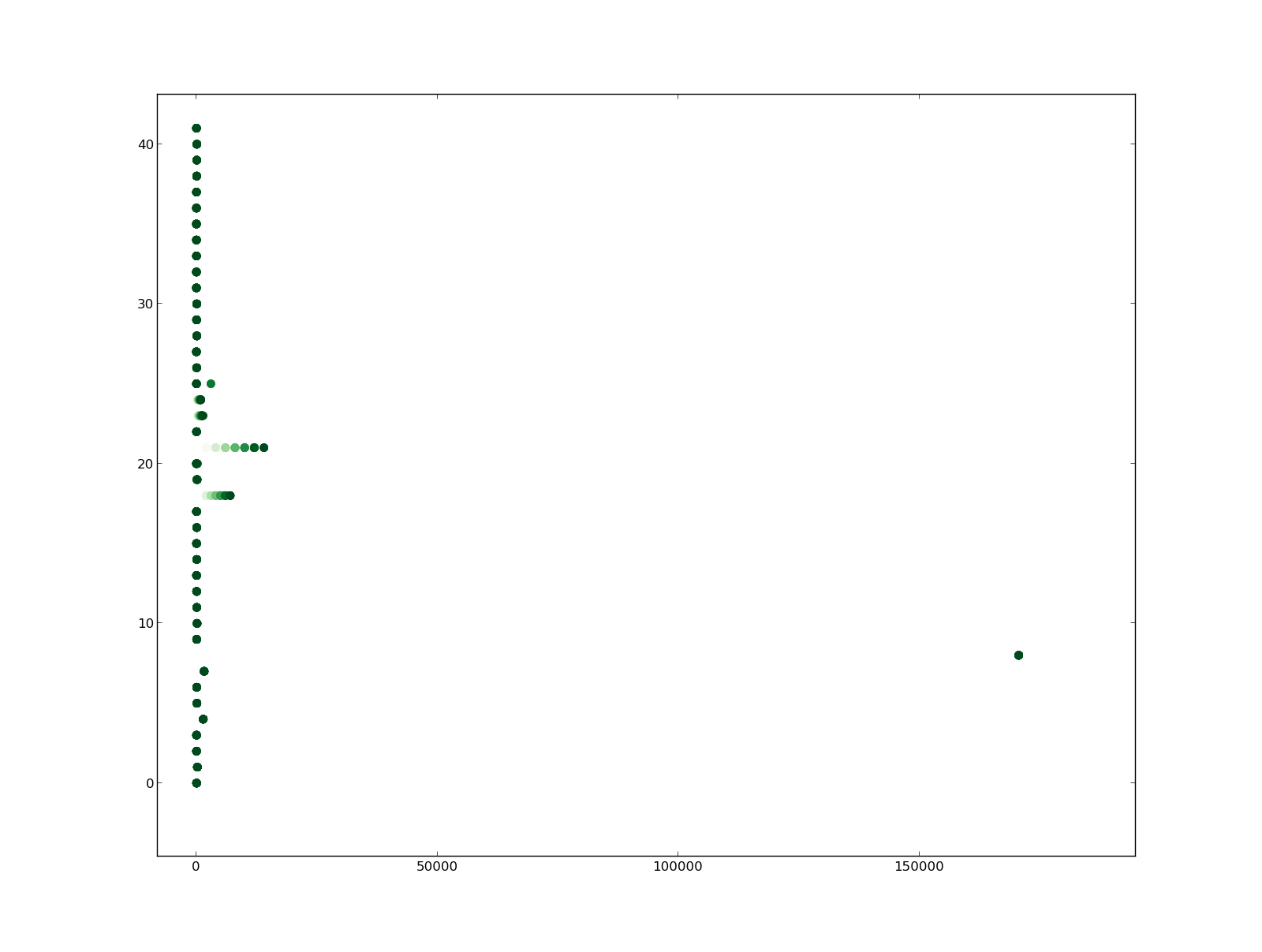
A logarithmic scale can be used instead of a linear one:
elplot.sizePlot(scale='log')
As said in a previous post, this allows to see both small and big memory leaks immediately:
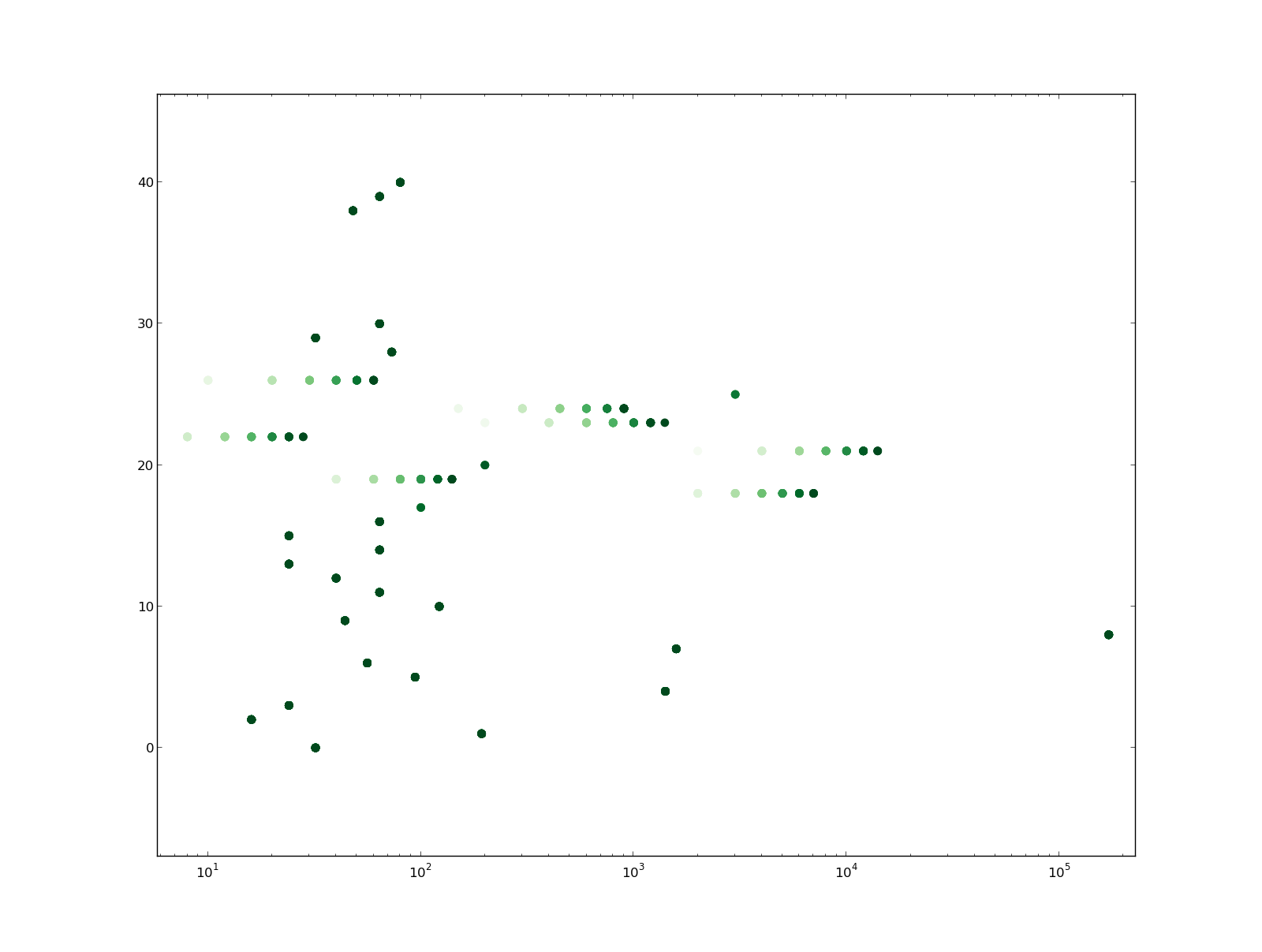
Retrieving Call Stacks
Within an asset, it is then very easy to walk through the allocers list, or inspect a specific item:
In [24]: asset.getAllocerList()[21] Out[24]: {u'id': 21, u'stack': [u'0x804886a:Leaker(unsigned int)']}
Next Steps
The availability of the python client does not mean that the html one is dead. However these two clients will probably evolve in different ways :
- The html client is probably more suited for live analysis and its GUI allows a lot of interactions.
- The python client is more suited to automate some tasks, and do things not directly available in the client.
However since prototyping with python is much faster than with html/js, chances are that the python client evolve faster than the html one.